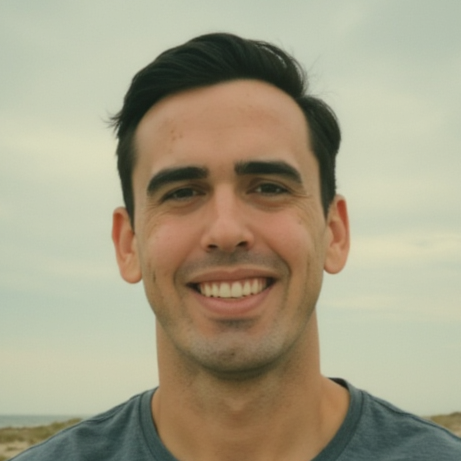
Computer Vision Engineer Interview Preparation
Overview of Qualifications for a Computer Vision Engineer
Educational Background
- Bachelor’s Degree: A degree in Computer Science, Electrical Engineering, Mathematics, or a related field is typically required. This provides a solid foundation in programming, algorithms, and data structures.
- Master’s/Ph.D.: Advanced degrees can be beneficial, particularly if they focus on computer vision, machine learning, or artificial intelligence. They provide deeper theoretical knowledge and research experience.
Certifications
- Deep Learning Specialization: Offered by Coursera in partnership with deeplearning.ai, this specialization covers neural networks, hyperparameter tuning, and more.
- Microsoft Certified: Azure AI Engineer Associate: This focuses on implementing AI solutions on Microsoft Azure, which includes computer vision applications.
- AWS Certified Machine Learning – Specialty: This certification validates expertise in building, training, and deploying machine learning models on AWS, including computer vision models.
Industry Qualifications
- Experience with Frameworks: Proficiency in TensorFlow, PyTorch, or OpenCV is often required. These are the most commonly used frameworks for developing computer vision applications.
- Familiarity with Computer Vision Libraries: Experience with libraries such as Scikit-image, PIL, or Dlib can be advantageous.
- Practical Experience: Hands-on experience through internships, projects, or previous positions is highly valued. Demonstrated ability to apply theoretical knowledge to solve real-world problems is critical.
- Publications and Contributions: Contributions to open-source projects or publications in relevant conferences/journals can greatly enhance a candidate’s profile.
Interview Questions and Answers
Technical Questions
What is the difference between supervised and unsupervised learning, and how do they apply to computer vision?
- Answer:
-
Supervised Learning: Involves training a model on a labeled dataset, meaning each training example is paired with an output label. Application: Object detection and classification tasks, such as identifying and classifying images of cats and dogs.
-
Unsupervised Learning: Involves training on data without labeled responses. The model tries to learn the patterns and structures from the data itself. Application: Image segmentation and clustering, such as grouping similar images together without predefined labels.
-
Example: For a facial recognition system:
- Supervised: Train a model to recognize faces using a labeled dataset with names.
- Unsupervised: Cluster faces based on facial features without knowing the identities.
-
Key Considerations:
- Supervised Learning: Requires a large amount of labeled data, which can be expensive and time-consuming to obtain.
- Unsupervised Learning: Useful when labels are unavailable, but it might not always produce interpretable results.
-
Pitfalls to Avoid:
- Supervised Learning: Overfitting to the training data if the model is too complex.
- Unsupervised Learning: Choosing the wrong clustering algorithm for the dataset can lead to poor results.
-
Follow-Up Points:
- Discuss scenarios where semi-supervised learning might be beneficial.
- Explain transfer learning’s role in reducing the need for labeled data.
-
How do convolutional neural networks (CNNs) work, and why are they well-suited for image processing tasks?
- Answer:
-
Convolutional Neural Networks (CNNs): Designed to process data with grid-like topology, such as images. They use convolutional layers to automatically and adaptively learn spatial hierarchies of features from input data.
-
Key Components:
- Convolutional Layers: Apply a set of filters to the input image, capturing spatial patterns.
- Pooling Layers: Reduce the spatial dimensions, retaining important features while reducing computation.
- Fully Connected Layers: Interpret the features learned by convolutional layers and output a classification decision.
-
Why CNNs for Images:
- Locality: Leverage the local connectivity pattern by focusing on local features.
- Parameter Sharing: Reduces the complexity and improves efficiency by using the same filter for different parts of the image.
- Translation Invariance: Pooling layers allow CNNs to recognize objects regardless of their position in the image.
-
Example:
- Image Classification: CNNs are widely used for tasks like detecting whether an image contains a cat or a dog.
-
Best Practices:
- Data Augmentation: Prevents overfitting by introducing varied data.
- Regularization Techniques: Such as dropout to prevent overfitting.
-
Pitfalls to Avoid:
- Overfitting: Especially with small datasets.
- Choosing Improper Architecture: Not all image tasks require deep networks.
-
Follow-Up Points:
- Discuss the role of different activation functions in CNNs.
- Compare CNNs with other architectures like RNNs for sequence data.
-
Behavioral Questions
Describe a challenging project you worked on. How did you handle the obstacles you faced?
- Answer:
-
Example: Developed a real-time object detection system for autonomous vehicles.
-
Challenges:
- Data Scarcity: Limited labeled data for training.
- Performance Constraints: High accuracy required under low latency.
-
Approach:
- Data Augmentation: Used techniques like flipping, rotation, and scaling to artificially increase the dataset size.
- Model Optimization: Implemented model pruning and quantization to meet performance constraints.
-
Outcome: Successfully deployed a system that met accuracy and latency requirements.
-
Key Considerations:
- Communication: Regular updates to stakeholders to manage expectations.
- Iteration: Constantly iterating on model design based on test results.
-
Pitfalls to Avoid:
- Ignoring Edge Cases: Failing to account for rare but critical scenarios.
- Over-Engineering: Adding unnecessary complexity can lead to maintenance challenges.
-
Follow-Up Points:
- Discuss how you balance technical depth with project deadlines.
- Share how you prioritize tasks when faced with multiple challenges.
-
Situational Questions
How would you approach a situation where a model you’ve deployed to production is underperforming?
- Answer:
- Initial Assessment:
- Log Analysis: Check logs for errors or anomalies in data input.
- Data Drift: Ensure the input data distribution hasn’t changed from the training data.
- Steps to Address:
- Model Retraining: If data drift is detected, update the dataset and retrain the model.
- Hyperparameter Tuning: Adjust hyperparameters to improve performance.
- Ensemble Methods: Combine predictions from multiple models to boost performance.
- Example:
- Scenario: A facial recognition system’s accuracy dropped after deployment.
- Solution: Discovered data drift due to a new lighting condition. Retrained the model with additional images accounting for the new conditions.
- Outcome: Restored accuracy to the expected levels.
- Key Considerations:
- Monitoring: Implement continuous monitoring to catch performance degradation early.
- Version Control: Maintain versions of models and datasets for traceability.
- Pitfalls to Avoid:
- Quick Fixes: Avoid making changes without understanding the root cause.
- Ignoring User Feedback: Valuable insights can be gained from end-users experiencing issues.
- Follow-Up Points:
- Discuss the importance of A/B testing in evaluating model changes.
- Explain how you ensure minimal downtime during model updates.
- Initial Assessment:
Problem-Solving Questions
Imagine you are tasked with designing a system to automatically tag images in a social media app. How would you approach this problem?
- Answer:
- Problem Definition:
- Objective: Automatically tag images with relevant labels.
- Constraints: High accuracy, low latency, and ability to handle a diverse set of images.
- Design Approach:
- Data Collection: Gather a large, diverse, labeled dataset for training.
- Model Selection: Use a pre-trained CNN (e.g., ResNet) with transfer learning to adapt to specific tags.
- System Architecture: Implement a scalable microservices architecture for handling high user volume.
- Example:
- Scenario: Instagram-like app requiring automatic tagging.
- Solution: Leveraged a transfer learning approach with InceptionV3, fine-tuned on the app’s specific dataset.
- Outcome: Achieved high accuracy and reduced the need for manual tagging.
- Key Considerations:
- User Feedback Loop: Implement a mechanism for users to correct tags and improve model performance.
- Scalability: Ensure the system can handle peak loads.
- Pitfalls to Avoid:
- Overfitting: Regularly validate the model on unseen data.
- Ignoring Diversity: Ensure the dataset represents the diversity of images the app will encounter.
- Follow-Up Points:
- Discuss the role of unsupervised learning to discover new tags.
- Explore ethical considerations in automated tagging, such as bias and privacy concerns.
- Problem Definition:
This guide should serve as a comprehensive resource for preparing for a computer vision engineer interview, covering a wide range of questions and detailed answers that demonstrate technical proficiency and problem-solving capabilities.
More Data Science Interview Guides
Explore more interview guides for Technical positions.
Feature Engineer Interview Help
The Feature Engineer Interview Help guide equips job seekers with essential skills and insights to excel in interview...
Senior Data Scientist Interview Questions and Answers
This guide offers comprehensive insights into the Senior Data Scientist interview process, equipping job seekers with...
Data Engineer Interview Preparation
This Data Engineer Interview Preparation guide equips job seekers with the skills and knowledge needed to excel in in...
Data Governance Specialist Interview Questions and Answers
This guide offers a comprehensive collection of Data Governance Specialist interview questions and answers designed t...
Machine Learning Engineer Interview Help
Unlock the secrets to acing your machine learning engineer interview with this comprehensive guide. Discover key topi...
Recent Blog Articles
Check out recent articles from Tustin Recruiting on all things hiring.
How to Implement Structured JSON-LD for Google Jobs
Learn how to implement structured JSON-LD for Google Jobs to improve your job postings and attract more qualified can...
Common Employee Benefits in Orange County, CA Private Sector
Discover common employee benefits offered by private sector employers in Orange County, CA.
10 High-Paying Sales Jobs You Can Get Without a Degree
Discover 10 high-paying sales jobs you can get without a degree, including entry-level roles and opportunities for ca...
When to Follow Up with a Recruiter
Learn when to follow up with a recruiter after submitting your resume and when to wait for best practices.
Exceptional Software Engineer Jobs in Orange County
Discover top software engineer jobs in Orange County. Unlock salary insights, skills needed, and career tips.
Featured Jobs
-
- Company
- Tustin Recruiting
- Title and Location
- Account Executive Equipment Finance
- Irvine, CA
- Employment Type
- FULL_TIME
- Salary
- $75,000-$95,000/YEAR
- Team and Date
- Equipment Finance
- Posted: 02/09/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Account Executive Equipment Finance
- Anaheim Hills, CA
- Employment Type
- FULL_TIME
- Salary
- $75,000-$95,000/YEAR
- Team and Date
- Equipment Finance
- Posted: 02/09/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Junior Account Executive
- Hayward, CA
- Employment Type
- FULL_TIME
- Salary
- $62,330-$79,329/YEAR
- Team and Date
- Software
- Posted: 01/29/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Sales Operations Coordinator
- Eugene, OR
- Employment Type
- FULL_TIME
- Salary
- $45,156-$58,201/YEAR
- Team and Date
- Software
- Posted: 01/29/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Account Executive
- Cypress, TX
- Employment Type
- FULL_TIME
- Salary
- $55,000-$70,000/YEAR
- Team and Date
- Equipment Finance
- Posted: 01/29/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Mobile App Developer
- Lakewood, CA
- Employment Type
- FULL_TIME
- Salary
- $85,013-$118,074/YEAR
- Team and Date
- Software
- Posted: 01/29/2025
Ready to find your next great hire?
Let's discuss your hiring needs. With our deep Orange County network and 20+ years of experience, we'll help you find the perfect candidate.
20+ Years Experience
Deep expertise and a proven track record of successful placements.
Direct-Hire Focus
Specialized in permanent placements that strengthen your team for the long term.
Local Market Knowledge
Unmatched understanding of Orange County's talent landscape and salary expectations.
Premium Job Board
Access top Orange County talent through our curated job board focused on quality over quantity.
Tustin Recruiting is for Everyone
At Tustin Recruiting, we are dedicated to fostering an inclusive environment that values diverse perspectives, ideas, and backgrounds. We strive to ensure equal employment opportunities for all applicants and employees. Our commitment is to prevent discrimination based on any protected characteristic, including race, color, ancestry, national origin, religion, creed, age, disability (mental and physical), sex, gender, sexual orientation, gender identity, gender expression, medical condition, genetic information, family care or medical leave status, marital status, domestic partner status, and military and veteran status.
We uphold all characteristics protected by US federal, state, and local laws, as well as the laws of the country or jurisdiction where you work.