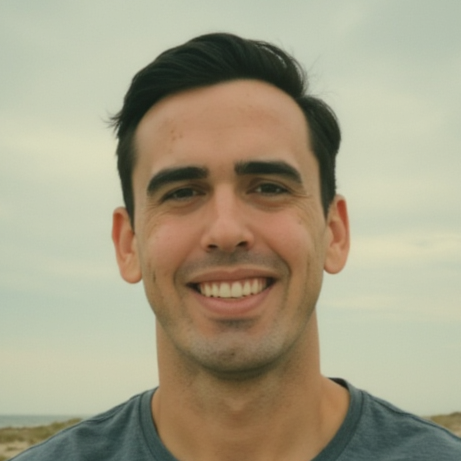
Front-End Engineer Interview Preparation
Front-End Engineer Interview Preparation Guide
Overview of Required and Recommended Certifications, Educational Background, and Industry Qualifications
To excel as a Front-End Engineer, candidates should possess a blend of educational qualifications, certifications, and industry experience. Here’s a brief overview:
-
Educational Background:
- Bachelor’s Degree: A degree in Computer Science, Software Engineering, or a related field is often preferred.
- Relevant Coursework: Web development, computer programming, data structures, and algorithms.
-
Certifications:
- Certified Web Developer: Offered by various institutions, covering HTML, CSS, JavaScript, and web design principles.
- JavaScript Certifications: Such as those from freeCodeCamp or JavaScript Mastery.
- React, Angular, or VueJS Certifications: Prove expertise in popular front-end frameworks.
-
Industry Qualifications:
- Portfolio of Work: Demonstrates practical experience and a range of completed projects.
- Open Source Contributions: Active participation in open source projects can enhance credibility.
- Experience with Version Control Systems: Familiarity with Git and collaborative platforms like GitHub.
Interview Questions and Answers
Technical Questions
-
What is the DOM, and how do you manipulate it?
Answer:
- DOM Overview: The Document Object Model (DOM) is a programming interface for web documents. It represents the page so that programs can change the document structure, style, and content.
- Manipulation Techniques:
- JavaScript Methods:
document.getElementById()
,document.querySelector()
,element.appendChild()
,element.removeChild()
, etc. - Example: To change the text of a paragraph with id
myPara
:document.getElementById('myPara').innerText = 'New text';
- jQuery: Simplifies DOM manipulation with methods like
.html()
,.append()
, and.remove()
.
- JavaScript Methods:
- Best Practices:
- Minimize DOM manipulations for better performance.
- Use methods like
documentFragment
for batch updates.
- Pitfalls to Avoid:
- Directly manipulating the DOM in large applications without frameworks can lead to performance issues.
- Follow-up Points:
- Discuss how modern frameworks like React use virtual DOM to optimize updates.
-
Explain the difference between
==
and===
in JavaScript.Answer:
==
: Compares two values for equality after converting both values to a common type (type coercion).- Example:
5 == '5'
istrue
because the string'5'
is converted to a number. ===
: Strict equality comparison. It checks for equality without type conversion.- Example:
5 === '5'
isfalse
because the types are different. - Best Practices:
- Prefer
===
to avoid unexpected results due to type coercion.
- Prefer
- Pitfalls to Avoid:
- Using
==
can lead to hard-to-debug errors due to implicit type conversion.
- Using
- Follow-up Points:
- Discuss scenarios where
==
might be intentionally used.
- Discuss scenarios where
-
What are CSS preprocessors, and why would you use them?
Answer:
- Overview: CSS preprocessors extend CSS with variables, nesting, and functions. Popular preprocessors include SASS, LESS, and Stylus.
- Benefits:
- Variables: Allow reuse of values throughout the stylesheet.
- Nesting: Makes CSS more readable and maintainable.
- Mixins and Functions: Enable code reuse and reduce redundancy.
- Example:
- SASS Variable:
$primary-color: #333; body { color: $primary-color; }
- SASS Variable:
- Best Practices:
- Use preprocessors to maintain large and complex stylesheets.
- Avoid over-nesting as it can lead to specificity issues.
- Pitfalls to Avoid:
- Not compiling the preprocessor files correctly can lead to missing styles.
- Follow-up Points:
- Discuss the role of CSS-in-JS libraries in modern front-end development.
Behavioral Questions
-
Describe a time when you had to work under pressure.
Answer:
- Context: While working on a project that had a tight deadline, the client requested significant changes at the last minute.
- Action: Prioritized tasks by impact, collaborated with team members to delegate responsibilities, and communicated regularly with the client to manage expectations.
- Outcome: Successfully implemented changes without compromising the project timeline or quality.
- Best Practices:
- Prioritize tasks based on urgency and importance.
- Maintain open communication with stakeholders.
- Pitfalls to Avoid:
- Avoid working in isolation; leverage team resources.
- Follow-up Points:
- Discuss strategies to handle similar situations in future projects.
-
How do you handle constructive criticism?
Answer:
- Approach:
- Listen actively and without defensiveness.
- Ask clarifying questions if needed.
- Reflect on the feedback and identify actionable steps.
- Example: Received feedback about complex code readability. Improved by adopting clearer naming conventions and adding comments.
- Outcome: Enhanced code quality and team collaboration.
- Best Practices:
- View criticism as an opportunity for growth.
- Implement changes and seek further feedback.
- Pitfalls to Avoid:
- Avoid taking criticism personally or becoming defensive.
- Follow-up Points:
- Discuss a specific instance where feedback led to a significant positive change.
- Approach:
Situational Questions
-
If a project deadline is approaching and you are behind schedule, what steps would you take?
Answer:
- Assess the Situation: Evaluate the remaining tasks and identify bottlenecks.
- Prioritize Tasks: Focus on high-impact deliverables.
- Communicate: Inform stakeholders of the situation and negotiate deadline extensions if possible.
- Leverage Team Resources: Delegate tasks to team members with available bandwidth.
- Outcome: Successfully delivered the core features on time while negotiating additional time for non-critical features.
- Best Practices:
- Maintain transparency with stakeholders.
- Prioritize tasks effectively.
- Pitfalls to Avoid:
- Avoid last-minute rushes that compromise quality.
- Follow-up Points:
- Discuss how you would apply lessons learned to future projects.
-
Imagine you are asked to work with a new framework you’re unfamiliar with. How would you approach this task?
Answer:
- Research: Start by understanding the framework’s core concepts and documentation.
- Hands-on Practice: Build a small project or component using the framework.
- Leverage Online Resources: Utilize tutorials, forums, and community support.
- Collaborate: Seek guidance from colleagues or mentors experienced with the framework.
- Outcome: Gained proficiency and successfully integrated the framework into the project.
- Best Practices:
- Embrace continuous learning and adaptability.
- Pitfalls to Avoid:
- Avoid diving into complex features without understanding the basics.
- Follow-up Points:
- Discuss how this approach has helped in past learning experiences.
Problem-solving Questions
-
How would you optimize a web page to improve load time?
Answer:
- Minimize HTTP Requests: Reduce the number of elements like scripts, images, and CSS files.
- Use Asynchronous Loading: Load JavaScript asynchronously using
async
ordefer
. - Optimize Images: Compress images and use modern formats like WebP.
- Leverage Browser Caching: Set appropriate cache-control headers.
- Minification and Bundling: Minify CSS and JavaScript and bundle them to reduce file size.
- Example: Implemented lazy loading for images, reducing initial page load time by 30%.
- Best Practices:
- Conduct regular performance audits using tools like Lighthouse.
- Pitfalls to Avoid:
- Avoid overly aggressive caching that may deliver outdated content.
- Follow-up Points:
- Discuss the impact of performance optimization on user engagement and SEO.
-
How would you handle a situation where a webpage works on desktop but not on mobile?
Answer:
- Identify the Issue: Use developer tools to inspect the mobile version and identify discrepancies.
- Responsive Design: Ensure the use of responsive design techniques like media queries and flexible layouts.
- Test Across Devices: Test the webpage on multiple devices and browsers.
- Example: Discovered a fixed-width element causing layout issues on mobile; resolved by using percentage-based widths.
- Outcome: Achieved a consistent and functional design across all devices.
- Best Practices:
- Adopt a mobile-first design approach.
- Pitfalls to Avoid:
- Avoid assuming desktop solutions will automatically work on mobile.
- Follow-up Points:
- Discuss tools and techniques for responsive design testing and validation.
Additional Questions
-
Explain the concept of closures in JavaScript.
-
Describe a challenging project and how you managed to complete it successfully.
-
How do you stay updated with the latest front-end technologies and trends?
-
What strategies do you use for debugging front-end applications?
-
Can you describe the difference between block, inline, and inline-block elements in CSS?
-
How do you ensure the accessibility of a web application?
-
Describe a time when you disagreed with a team member and how you resolved it.
-
What is the purpose of REST APIs, and how do you consume them in a front-end application?
-
How would you handle a feature request that conflicts with the current project scope?
-
Explain the importance of version control in collaborative projects.
-
What are CSS Flexbox and Grid, and when would you use each?
This comprehensive guide covers various aspects of front-end engineering, providing candidates with a solid foundation for acing their interviews. By understanding these concepts, preparing for potential questions, and learning from real-world examples, candidates can demonstrate their expertise and readiness for the role.
More Software Engineering Interview Guides
Explore more interview guides for Technical positions.
AI Engineer Interview Preparation
The AI Engineer Interview Preparation guide equips job seekers with essential skills and knowledge to excel in AI eng...
Back-End Developer Interview Help
Back-End Developer Interview Help equips job seekers with essential strategies and insights to ace their interviews. ...
Mobile App Developer Interview Help
The Mobile App Developer Interview Help guide equips job seekers with essential strategies to excel in interviews. Le...
Blockchain Developer Interview Questions and Answers
This guide offers a comprehensive collection of Blockchain Developer interview questions and answers, designed to hel...
Software Architect Interview Questions and Answers
Discover essential insights with our Software Architect Interview Questions and Answers guide. Tailored for job seeke...
Recent Blog Articles
Check out recent articles from Tustin Recruiting on all things hiring.
How to Implement Structured JSON-LD for Google Jobs
Learn how to implement structured JSON-LD for Google Jobs to improve your job postings and attract more qualified can...
Common Employee Benefits in Orange County, CA Private Sector
Discover common employee benefits offered by private sector employers in Orange County, CA.
10 High-Paying Sales Jobs You Can Get Without a Degree
Discover 10 high-paying sales jobs you can get without a degree, including entry-level roles and opportunities for ca...
When to Follow Up with a Recruiter
Learn when to follow up with a recruiter after submitting your resume and when to wait for best practices.
Exceptional Software Engineer Jobs in Orange County
Discover top software engineer jobs in Orange County. Unlock salary insights, skills needed, and career tips.
Featured Jobs
-
- Company
- Tustin Recruiting
- Title and Location
- Account Executive Equipment Finance
- Irvine, CA
- Employment Type
- FULL_TIME
- Salary
- $75,000-$95,000/YEAR
- Team and Date
- Equipment Finance
- Posted: 02/09/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Account Executive Equipment Finance
- Anaheim Hills, CA
- Employment Type
- FULL_TIME
- Salary
- $75,000-$95,000/YEAR
- Team and Date
- Equipment Finance
- Posted: 02/09/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Junior Account Executive
- Hayward, CA
- Employment Type
- FULL_TIME
- Salary
- $62,330-$79,329/YEAR
- Team and Date
- Software
- Posted: 01/29/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Sales Operations Coordinator
- Eugene, OR
- Employment Type
- FULL_TIME
- Salary
- $45,156-$58,201/YEAR
- Team and Date
- Software
- Posted: 01/29/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Account Executive
- Cypress, TX
- Employment Type
- FULL_TIME
- Salary
- $55,000-$70,000/YEAR
- Team and Date
- Equipment Finance
- Posted: 01/29/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Mobile App Developer
- Lakewood, CA
- Employment Type
- FULL_TIME
- Salary
- $85,013-$118,074/YEAR
- Team and Date
- Software
- Posted: 01/29/2025
Ready to find your next great hire?
Let's discuss your hiring needs. With our deep Orange County network and 20+ years of experience, we'll help you find the perfect candidate.
20+ Years Experience
Deep expertise and a proven track record of successful placements.
Direct-Hire Focus
Specialized in permanent placements that strengthen your team for the long term.
Local Market Knowledge
Unmatched understanding of Orange County's talent landscape and salary expectations.
Premium Job Board
Access top Orange County talent through our curated job board focused on quality over quantity.
Tustin Recruiting is for Everyone
At Tustin Recruiting, we are dedicated to fostering an inclusive environment that values diverse perspectives, ideas, and backgrounds. We strive to ensure equal employment opportunities for all applicants and employees. Our commitment is to prevent discrimination based on any protected characteristic, including race, color, ancestry, national origin, religion, creed, age, disability (mental and physical), sex, gender, sexual orientation, gender identity, gender expression, medical condition, genetic information, family care or medical leave status, marital status, domestic partner status, and military and veteran status.
We uphold all characteristics protected by US federal, state, and local laws, as well as the laws of the country or jurisdiction where you work.