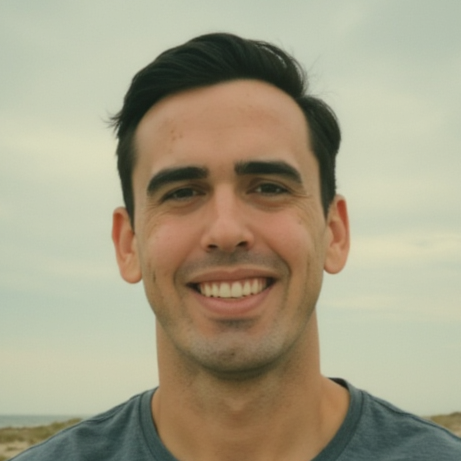
Mobile App Developer Interview Help
Overview of Required and Recommended Certifications, Educational Background, and Industry Qualifications
Educational Background
- Bachelor’s Degree in Computer Science or Related Field: A solid foundation in computer science principles, algorithms, and data structures is essential. Courses in software engineering, mobile app development, and user interface design are highly beneficial.
- Master’s Degree: While not always necessary, a master’s degree in computer science, software engineering, or a related field can be advantageous for advanced positions or leadership roles.
Certifications
- Certified Mobile App Developer (CMAD): This certification is recognized in the industry and covers a broad spectrum of app development, including design, development, and deployment.
- Google Associate Android Developer: Specifically for Android developers, this certification demonstrates competency in Android development and familiarity with Android Studio, APIs, and other tools.
- Apple Certified iOS App Developer: For iOS developers, this certification validates proficiency in developing apps for Apple’s ecosystem using Swift and Xcode.
- AWS Certified Developer: As cloud integration becomes more prevalent, having a certification in AWS can be beneficial for developers working with cloud-based applications.
Industry Qualifications
- Experience with Mobile App Frameworks: Proficiency in frameworks such as React Native, Flutter, or Xamarin is often required.
- Portfolio of Published Apps: Demonstrating experience through a portfolio of apps available in app stores can significantly enhance a candidate’s profile.
- GitHub Contributions: Active contributions to open source projects or a well-maintained GitHub repository can showcase practical skills and collaboration.
- Agile and Scrum Methodologies: Familiarity with Agile development processes and experience working in Scrum teams are often valued.
Interview Questions
Technical Questions
1. What are the differences between native, hybrid, and web apps, and when would you choose each?
Answer:
-
Native Apps: Developed specifically for one platform (iOS or Android) using platform-specific languages (Swift/Objective-C for iOS, Java/Kotlin for Android).
- Pros: High performance, access to device features, better user experience.
- Cons: Higher cost and longer development time.
- When to choose: When performance and user experience are top priorities, and there’s a budget to maintain separate codebases.
- Scenario: A gaming app that requires high frame rates and intensive graphics rendering is best suited for native development.
-
Hybrid Apps: Built using web technologies (HTML, CSS, JavaScript) and wrapped in a native shell.
- Pros: Faster development, single codebase for multiple platforms.
- Cons: Compromised performance and potential UI/UX issues.
- When to choose: When time-to-market is crucial and the app does not require intensive hardware usage.
- Scenario: A content delivery app that needs to be available quickly across multiple platforms with minimal platform-specific features.
-
Web Apps: Optimized websites that look and feel like apps.
- Pros: No need for app store distribution, easier maintenance.
- Cons: Limited access to device features, dependent on browser compatibility.
- When to choose: For apps that do not require offline capabilities or access to device sensors.
- Scenario: A simple task management tool that users can access through any device’s browser without installation.
Follow-Up Points:
- Discuss specific tools and frameworks like React Native or Flutter for hybrid apps.
- Consideration of Progressive Web Apps (PWAs) as a modern alternative.
2. How do you handle different screen sizes and orientations in mobile app design?
Answer:
- Responsive Design: Use flexible layouts and scalable units (e.g., percentages, em units) instead of fixed sizes.
- Example: Designing a grid layout using CSS Flexbox to adjust automatically based on the screen size.
- Adaptive Design: Create different layouts for different devices or orientations explicitly.
- Example: Using
size classes
in iOS to provide specific layouts for compact and regular screens.
- Example: Using
- Auto Layout (iOS) and Constraint Layout (Android): Utilize these systems to define relationships between views that adapt to screen changes.
- Example: Setting constraints in Android’s XML layout files to ensure components resize and reposition on different devices.
- Vector Graphics: Use vector graphics (e.g., SVGs) to ensure images scale without losing quality.
- Example: Utilizing vector drawables in Android to maintain quality across different resolutions.
Best Practices:
- Testing on Multiple Devices: Regular testing on a variety of devices to ensure consistent experience.
- Design Tools: Tools like Figma or Adobe XD for creating responsive and adaptive design prototypes.
Common Pitfalls:
- Ignoring edge cases for extreme screen sizes.
- Over-reliance on third-party libraries without customization.
Follow-Up Points:
- Discuss any recent projects involving responsive design challenges.
- Explain how to optimize performance alongside responsive design.
Behavioral Questions
3. Describe a time when you faced a conflict in a team project. How did you handle it?
Answer:
- Situation: During a mobile app development project, there was a disagreement between the design and development teams regarding the feasibility of certain UI components.
- Task: As the lead developer, it was my responsibility to mediate the conflict and ensure project timelines were met without compromising the app’s functionality.
- Action: I organized a meeting with both teams to facilitate open communication. We listed the concerns of both sides and prioritized the most critical UI components. I demonstrated how some features could be modified with minimal impact on design fidelity while significantly reducing development complexity.
- Outcome: The teams agreed on a compromise that maintained the app’s aesthetic appeal while adhering to the project timeline. This not only resolved the conflict but also improved inter-team collaboration.
Alternate Scenario:
- Situation: A team member was consistently missing deadlines, affecting the project flow.
- Action: I approached the team member to understand their challenges and discovered they were struggling with a specific technology. I arranged for a mentoring session with a more experienced developer.
- Outcome: The team member’s performance improved, and the project was back on track.
Best Practices:
- Active Listening: Understand all perspectives before proposing solutions.
- Facilitation Skills: Lead discussions to promote constructive dialogue.
Common Pitfalls:
- Avoiding confrontation and not addressing conflicts early on.
- Imposing solutions without team consensus.
Follow-Up Points:
- How to foster an environment that minimizes conflicts.
- Strategies for continuous team alignment.
Situational Questions
4. If a critical bug is reported in production, what steps would you take to address it?
Answer:
- Immediate Assessment: Determine the severity and impact of the bug. If it affects a core functionality, prioritize it over other tasks.
- Example: A payment processing bug in an e-commerce app would be critical due to potential revenue loss.
- Reproduction: Attempt to reproduce the bug in a controlled environment to understand the issue.
- Example: Use logs, error reports, and user feedback to pinpoint the bug.
- Temporary Patch: Implement a quick fix if possible to mitigate immediate impact while working on a permanent solution.
- Example: Rolling back to a previous stable version if the bug was introduced in a recent update.
- Root Cause Analysis: Investigate the underlying cause to prevent recurrence.
- Example: Conduct a code review to identify where the process failed.
- Deployment: Once fixed, deploy the update and monitor for any related issues.
- Example: Use feature flags to enable the fix for a small user segment first.
Best Practices:
- Communication: Keep stakeholders informed about the bug status and resolution timeline.
- Documentation: Update documentation with the bug details and resolution steps.
Common Pitfalls:
- Rushing to fix without understanding the full impact.
- Lack of communication with users and stakeholders.
Follow-Up Points:
- Discuss a specific instance of a critical bug you resolved.
- How to ensure quality assurance to minimize production bugs.
Problem-Solving Questions
5. How would you optimize the performance of a mobile app?
Answer:
- Code Optimization: Refactor code to enhance efficiency and reduce redundancy.
- Example: Optimize loops and conditional statements to lower computational overhead.
- Memory Management: Efficiently manage resources to prevent memory leaks.
- Example: Use weak references in Android to avoid retaining unnecessary objects.
- Asynchronous Processing: Use asynchronous tasks to handle intensive operations off the main thread.
- Example: Implementing Kotlin Coroutines for network requests in Android.
- Lazy Loading: Load resources only when they are needed to reduce initial load times.
- Example: Lazy loading images using libraries like Glide or Picasso.
- Profiling Tools: Use profiling tools to identify bottlenecks.
- Example: Android Profiler and Xcode Instruments to monitor CPU, memory, and network usage.
Best Practices:
- Testing Under Load: Simulate high-traffic conditions to ensure stability.
- User Feedback: Collect and analyze user feedback for performance-related issues.
Common Pitfalls:
- Over-optimization leading to code complexity.
- Neglecting user experience in favor of pure performance metrics.
Follow-Up Points:
- Discuss tools and metrics used for performance monitoring.
- How to balance performance optimization with feature development.
Conclusion
This guide provides a comprehensive approach to preparing for a mobile app developer interview. Candidates should focus on both technical skills and soft skills such as communication and problem-solving. Real-world scenarios and detailed answers will help demonstrate expertise and adaptability in various situations.
More Software Engineering Interview Guides
Explore more interview guides for Technical positions.
AI Engineer Interview Preparation
The AI Engineer Interview Preparation guide equips job seekers with essential skills and knowledge to excel in AI eng...
Back-End Developer Interview Help
Back-End Developer Interview Help equips job seekers with essential strategies and insights to ace their interviews. ...
Mobile App Developer Interview Help
The Mobile App Developer Interview Help guide equips job seekers with essential strategies to excel in interviews. Le...
Blockchain Developer Interview Questions and Answers
This guide offers a comprehensive collection of Blockchain Developer interview questions and answers, designed to hel...
Software Architect Interview Questions and Answers
Discover essential insights with our Software Architect Interview Questions and Answers guide. Tailored for job seeke...
Recent Blog Articles
Check out recent articles from Tustin Recruiting on all things hiring.
How to Implement Structured JSON-LD for Google Jobs
Learn how to implement structured JSON-LD for Google Jobs to improve your job postings and attract more qualified can...
Common Employee Benefits in Orange County, CA Private Sector
Discover common employee benefits offered by private sector employers in Orange County, CA.
10 High-Paying Sales Jobs You Can Get Without a Degree
Discover 10 high-paying sales jobs you can get without a degree, including entry-level roles and opportunities for ca...
When to Follow Up with a Recruiter
Learn when to follow up with a recruiter after submitting your resume and when to wait for best practices.
Exceptional Software Engineer Jobs in Orange County
Discover top software engineer jobs in Orange County. Unlock salary insights, skills needed, and career tips.
Featured Jobs
-
- Company
- Tustin Recruiting
- Title and Location
- Account Executive Equipment Finance
- Irvine, CA
- Employment Type
- FULL_TIME
- Salary
- $75,000-$95,000/YEAR
- Team and Date
- Equipment Finance
- Posted: 02/09/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Account Executive Equipment Finance
- Anaheim Hills, CA
- Employment Type
- FULL_TIME
- Salary
- $75,000-$95,000/YEAR
- Team and Date
- Equipment Finance
- Posted: 02/09/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Junior Account Executive
- Hayward, CA
- Employment Type
- FULL_TIME
- Salary
- $62,330-$79,329/YEAR
- Team and Date
- Software
- Posted: 01/29/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Sales Operations Coordinator
- Eugene, OR
- Employment Type
- FULL_TIME
- Salary
- $45,156-$58,201/YEAR
- Team and Date
- Software
- Posted: 01/29/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Account Executive
- Cypress, TX
- Employment Type
- FULL_TIME
- Salary
- $55,000-$70,000/YEAR
- Team and Date
- Equipment Finance
- Posted: 01/29/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Mobile App Developer
- Lakewood, CA
- Employment Type
- FULL_TIME
- Salary
- $85,013-$118,074/YEAR
- Team and Date
- Software
- Posted: 01/29/2025
Ready to find your next great hire?
Let's discuss your hiring needs. With our deep Orange County network and 20+ years of experience, we'll help you find the perfect candidate.
20+ Years Experience
Deep expertise and a proven track record of successful placements.
Direct-Hire Focus
Specialized in permanent placements that strengthen your team for the long term.
Local Market Knowledge
Unmatched understanding of Orange County's talent landscape and salary expectations.
Premium Job Board
Access top Orange County talent through our curated job board focused on quality over quantity.
Tustin Recruiting is for Everyone
At Tustin Recruiting, we are dedicated to fostering an inclusive environment that values diverse perspectives, ideas, and backgrounds. We strive to ensure equal employment opportunities for all applicants and employees. Our commitment is to prevent discrimination based on any protected characteristic, including race, color, ancestry, national origin, religion, creed, age, disability (mental and physical), sex, gender, sexual orientation, gender identity, gender expression, medical condition, genetic information, family care or medical leave status, marital status, domestic partner status, and military and veteran status.
We uphold all characteristics protected by US federal, state, and local laws, as well as the laws of the country or jurisdiction where you work.