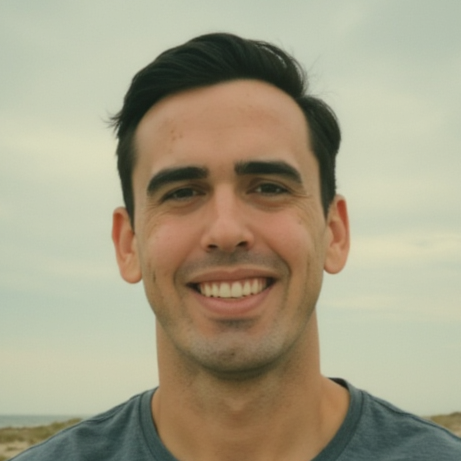
Full-Stack Developer Interview Preparation
Full-Stack Developer Interview Preparation Guide
Overview of Required and Recommended Certifications, Educational Background, and Industry Qualifications
A strong candidate for a Full-Stack Developer position typically possesses a combination of formal education, certifications, and industry experience. Here’s a breakdown of what can enhance a candidate’s profile:
-
Educational Background:
- Bachelor’s Degree in Computer Science or Related Field: Most positions require at least a bachelor’s degree in computer science, information technology, or a related field.
- Master’s Degree: While not always necessary, a master’s degree can be beneficial for more advanced positions or leadership roles.
-
Certifications:
- Certified Web Developer: Offered by various organizations, this certification validates a candidate’s understanding of web development principles.
- Microsoft Certified: Azure Developer Associate: Useful for roles involving cloud services.
- AWS Certified Developer – Associate: Important for roles involving Amazon Web Services.
- Full-Stack Web Development with React Specialization: Offered by Coursera, it covers React, Node.js, and MongoDB.
- Google Professional Cloud Developer: For roles focused on Google Cloud Platform.
-
Industry Qualifications:
- Experience with Popular Frameworks and Languages: Proficiency in JavaScript, Python, Ruby, PHP, and frameworks like React, Angular, and Vue.js.
- Version Control Systems (Git): Proficiency in using Git for version control.
- Database Management: Experience with SQL and NoSQL databases (e.g., MySQL, MongoDB).
- DevOps Knowledge: Understanding of CI/CD pipelines, Docker, and Kubernetes.
Interview Questions and Comprehensive Answers
Technical Questions
- What is the difference between REST and GraphQL?
Answer:
-
REST (Representational State Transfer):
- Structure: Based on stateless, client-server communication, primarily over HTTP.
- Data Fetching: Retrieves a fixed set of data; often requires multiple endpoints.
- Example: GET /api/users/123 retrieves user data with ID 123.
-
GraphQL:
- Structure: A query language for APIs and runtime for executing those queries.
- Data Fetching: Allows clients to request exactly the data they need.
- Example: A single query might retrieve a user and their posts:
{ user(id: "123") { name posts { title } } }
-
Real-World Scenario:
- REST: Suitable for simple, well-defined API structures with limited data needs.
- GraphQL: Ideal when the client requires flexibility and efficiency in data fetching.
-
Pitfalls:
- REST: Over-fetching or under-fetching data due to fixed endpoints.
- GraphQL: Complexity in setting up and maintaining the schema.
-
Best Practices:
- REST: Use for simpler, stable API structures.
- GraphQL: Use when flexibility and client-specific queries are necessary.
-
Follow-Up Points:
- Discuss potential security implications of exposing complex queries in GraphQL.
- Consider performance impacts when choosing between REST and GraphQL.
- Explain the concept of closures in JavaScript. How are they used in real-world applications?
Answer:
- Concept:
- A closure is a function that retains access to its lexical scope, even when the function is executed outside that scope.
- Example:
function outerFunction() { let outerVariable = 'I am outside!'; function innerFunction() { console.log(outerVariable); // Accesses outerVariable } return innerFunction; } const myClosure = outerFunction(); myClosure(); // Logs: 'I am outside!'
- Real-World Scenarios:
- Data Encapsulation: Creating private variables in modules.
- Event Handlers: Maintaining state across asynchronous operations.
- Pitfalls:
- Memory Leaks: Unused closures can consume memory.
- Best Practices:
- Use closures to encapsulate state and avoid polluting the global scope.
- Follow-Up Points:
- Discuss how closures can be used to create factory functions or manage asynchronous code.
Behavioral Questions
- Describe a time when you had to work with a difficult team member. How did you handle the situation?
Answer:
- Scenario:
- Context: While working on a project, a team member consistently missed deadlines, impacting the project timeline.
- Action Taken: Initiated a private conversation to understand their challenges. Discovered they were struggling with personal issues affecting their work.
- Outcome: Offered support by redistributing workload temporarily and connecting them with management for further assistance.
- Alternative Approaches:
- If direct conversation was ineffective, escalate to management while maintaining professionalism.
- Best Practices:
- Address issues early to prevent escalation.
- Foster an environment of open communication and support.
- Pitfalls:
- Avoid confrontation in public settings; it can exacerbate tensions.
- Follow-Up Points:
- Discuss ways to improve team dynamics and prevent similar issues.
- How do you prioritize your tasks when working on multiple projects?
Answer:
- Approach:
- Context: Multiple projects with overlapping deadlines.
- Action Taken: Use a prioritization matrix (e.g., Eisenhower Box) to categorize tasks by urgency and importance.
- Outcome: Ensured critical tasks were completed on time, maintaining project quality.
- Real-World Scenario:
- Example: Implementing a new feature while fixing critical bugs. Prioritize bug fixes to maintain system stability.
- Best Practices:
- Regularly review and adjust priorities as project demands change.
- Communicate with stakeholders to align on priorities.
- Pitfalls:
- Avoid overcommitting and diluting focus, leading to reduced quality.
- Follow-Up Points:
- Discuss how to handle unexpected changes in project priorities.
Situational Questions
- You are tasked with redesigning an existing application that has poor user feedback. What steps do you take to initiate this process?
Answer:
- Approach:
- Step 1: Conduct a comprehensive review of user feedback to identify pain points.
- Step 2: Collaborate with UX/UI designers to create prototypes addressing user concerns.
- Step 3: Implement iterative testing with user groups for continuous feedback.
- Outcome: A redesigned application with improved user satisfaction and engagement.
- Real-World Scenario:
- Example: An e-commerce site with a cumbersome checkout process. Focus on streamlining steps and enhancing clarity.
- Best Practices:
- Engage users throughout the redesign process for valuable insights.
- Use A/B testing to validate design decisions.
- Pitfalls:
- Avoid making changes based solely on assumptions without user input.
- Follow-Up Points:
- Discuss methods for measuring the success of the redesign.
- A critical system feature is not performing well under load. What is your approach to resolving this issue?
Answer:
- Approach:
- Step 1: Perform load testing to identify bottlenecks in the system.
- Step 2: Analyze code and database queries for optimization opportunities.
- Step 3: Implement solutions such as caching or optimizing algorithms.
- Outcome: Improved system performance to handle peak load efficiently.
- Real-World Scenario:
- Example: An API endpoint with slow response times during high traffic. Optimize by adding caching layers and revising query logic.
- Best Practices:
- Regularly test system under simulated load conditions.
- Prioritize optimizations based on impact and feasibility.
- Pitfalls:
- Avoid premature optimizations without identifying root causes.
- Follow-Up Points:
- Discuss monitoring strategies to prevent future performance issues.
Problem-Solving Questions
- How would you design a URL shortener?
Answer:
- Design Approach:
- Step 1: Design a database schema to store original URLs and their shortened versions.
- Step 2: Implement a hash function to generate unique short codes.
- Step 3: Create a mechanism to redirect short URLs to their original counterparts.
- Outcome: A scalable URL shortener service with efficient redirection.
- Real-World Scenario:
- Example: Implementing a URL shortener for a marketing campaign to track link usage.
- Best Practices:
- Use reliable hashing algorithms to minimize collisions.
- Optimize database queries for fast lookup and storage.
- Pitfalls:
- Avoid using sequential IDs which can be easily guessed.
- Follow-Up Points:
- Discuss potential security measures to prevent malicious URL submissions.
- Explain how you would implement a search feature for a website with a large dataset.
Answer:
- Design Approach:
- Step 1: Choose a search infrastructure like Elasticsearch for efficient indexing and querying.
- Step 2: Design a schema that optimizes for search performance.
- Step 3: Implement features like faceted search, autocomplete, and relevance ranking.
- Outcome: A responsive and accurate search feature that enhances user experience.
- Real-World Scenario:
- Example: Implementing search on an e-commerce platform with millions of products.
- Best Practices:
- Regularly update indexes to reflect the most current data.
- Use caching to improve search query performance.
- Pitfalls:
- Avoid unoptimized queries that can lead to slow response times.
- Follow-Up Points:
- Discuss strategies for handling search queries with high concurrency.
- What strategies would you use to ensure the security of a full-stack application?
Answer:
- Strategies:
- Input Validation: Sanitize and validate all user inputs to prevent injection attacks.
- Authentication and Authorization: Implement robust mechanisms using industry standards like OAuth 2.0.
- Data Encryption: Use SSL/TLS for data in transit and strong encryption for sensitive data at rest.
- Outcome: A secure application that protects user data and system integrity.
- Real-World Scenario:
- Example: Implementing two-factor authentication for a banking application.
- Best Practices:
- Regularly update dependencies to patch known vulnerabilities.
- Conduct routine security audits and penetration testing.
- Pitfalls:
- Avoid hardcoding sensitive information like API keys in the codebase.
- Follow-Up Points:
- Discuss the role of security in the CI/CD pipeline to catch vulnerabilities early.
Additional Questions
-
Describe how you handle version control in a multi-developer environment.
-
How do you ensure code quality and maintainability in your projects?
-
What is your approach to integrating third-party APIs into an application?
-
Can you explain the MVC architecture and its benefits?
-
How do you handle error handling and logging in your applications?
-
What is your approach to testing full-stack applications?
-
How do you optimize the performance of web applications?
-
Describe a challenging technical problem you solved. How did you approach it?
-
How do you stay updated with the latest trends and technologies in web development?
-
What are your strategies for managing technical debt in a project?
-
Describe a time when you had to learn a new technology quickly. How did you manage it?
This guide provides a comprehensive overview of what to expect and how to prepare for a Full-Stack Developer interview, covering a wide range of questions and providing detailed insights into each.
More Software Engineering Interview Guides
Explore more interview guides for Technical positions.
AI Engineer Interview Preparation
The AI Engineer Interview Preparation guide equips job seekers with essential skills and knowledge to excel in AI eng...
Back-End Developer Interview Help
Back-End Developer Interview Help equips job seekers with essential strategies and insights to ace their interviews. ...
Mobile App Developer Interview Help
The Mobile App Developer Interview Help guide equips job seekers with essential strategies to excel in interviews. Le...
Blockchain Developer Interview Questions and Answers
This guide offers a comprehensive collection of Blockchain Developer interview questions and answers, designed to hel...
Software Architect Interview Questions and Answers
Discover essential insights with our Software Architect Interview Questions and Answers guide. Tailored for job seeke...
Recent Blog Articles
Check out recent articles from Tustin Recruiting on all things hiring.
How to Implement Structured JSON-LD for Google Jobs
Learn how to implement structured JSON-LD for Google Jobs to improve your job postings and attract more qualified can...
Common Employee Benefits in Orange County, CA Private Sector
Discover common employee benefits offered by private sector employers in Orange County, CA.
10 High-Paying Sales Jobs You Can Get Without a Degree
Discover 10 high-paying sales jobs you can get without a degree, including entry-level roles and opportunities for ca...
When to Follow Up with a Recruiter
Learn when to follow up with a recruiter after submitting your resume and when to wait for best practices.
Exceptional Software Engineer Jobs in Orange County
Discover top software engineer jobs in Orange County. Unlock salary insights, skills needed, and career tips.
Featured Jobs
-
- Company
- Tustin Recruiting
- Title and Location
- Account Executive Equipment Finance
- Irvine, CA
- Employment Type
- FULL_TIME
- Salary
- $75,000-$95,000/YEAR
- Team and Date
- Equipment Finance
- Posted: 02/09/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Account Executive Equipment Finance
- Anaheim Hills, CA
- Employment Type
- FULL_TIME
- Salary
- $75,000-$95,000/YEAR
- Team and Date
- Equipment Finance
- Posted: 02/09/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Junior Account Executive
- Hayward, CA
- Employment Type
- FULL_TIME
- Salary
- $62,330-$79,329/YEAR
- Team and Date
- Software
- Posted: 01/29/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Sales Operations Coordinator
- Eugene, OR
- Employment Type
- FULL_TIME
- Salary
- $45,156-$58,201/YEAR
- Team and Date
- Software
- Posted: 01/29/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Account Executive
- Cypress, TX
- Employment Type
- FULL_TIME
- Salary
- $55,000-$70,000/YEAR
- Team and Date
- Equipment Finance
- Posted: 01/29/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Mobile App Developer
- Lakewood, CA
- Employment Type
- FULL_TIME
- Salary
- $85,013-$118,074/YEAR
- Team and Date
- Software
- Posted: 01/29/2025
Ready to find your next great hire?
Let's discuss your hiring needs. With our deep Orange County network and 20+ years of experience, we'll help you find the perfect candidate.
20+ Years Experience
Deep expertise and a proven track record of successful placements.
Direct-Hire Focus
Specialized in permanent placements that strengthen your team for the long term.
Local Market Knowledge
Unmatched understanding of Orange County's talent landscape and salary expectations.
Premium Job Board
Access top Orange County talent through our curated job board focused on quality over quantity.
Tustin Recruiting is for Everyone
At Tustin Recruiting, we are dedicated to fostering an inclusive environment that values diverse perspectives, ideas, and backgrounds. We strive to ensure equal employment opportunities for all applicants and employees. Our commitment is to prevent discrimination based on any protected characteristic, including race, color, ancestry, national origin, religion, creed, age, disability (mental and physical), sex, gender, sexual orientation, gender identity, gender expression, medical condition, genetic information, family care or medical leave status, marital status, domestic partner status, and military and veteran status.
We uphold all characteristics protected by US federal, state, and local laws, as well as the laws of the country or jurisdiction where you work.