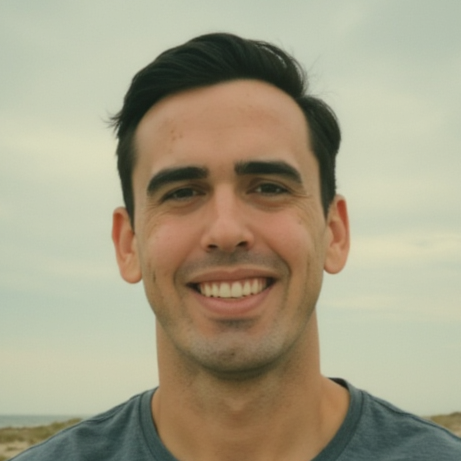
Java Developer Interview Help
Java Developer Interview Preparation Guide
Overview of Required and Recommended Certifications, Educational Background, and Industry Qualifications
To excel as a Java Developer, candidates should focus on building a strong educational foundation, obtaining relevant certifications, and gaining industry experience that highlights their skills and dedication to the field.
Educational Background
- Bachelor’s Degree in Computer Science or Related Field: Most Java Developer positions require a minimum of a bachelor’s degree in computer science, software engineering, or a related field. This provides foundational knowledge in programming, algorithms, data structures, and computer systems.
- Master’s Degree (Recommended): For advanced roles or specialized positions, a Master’s degree in Computer Science or Software Development can be advantageous.
Certifications
- Oracle Certified Professional Java SE Programmer (OCPJP): This certification demonstrates a high level of proficiency in Java programming and is highly regarded in the industry.
- Oracle Certified Master, Java EE Enterprise Architect (OCMJEA): Suitable for senior developers, this certification covers enterprise-level Java application architecture.
- Spring Professional Certification: Validates skills in using the Spring framework, which is widely used in enterprise Java applications.
- AWS Certified Developer – Associate: For Java developers looking to work in cloud environments, this certification demonstrates proficiency in AWS services, which is increasingly important.
Industry Qualifications
- Experience with Java Frameworks: Proficiency in frameworks such as Spring, Hibernate, and JavaServer Faces (JSF) is often required.
- Version Control Systems: Experience with Git or similar version control systems is essential for collaborative development.
- Agile Methodologies: Familiarity with Agile, Scrum, or Kanban methodologies is beneficial, as many development teams use these frameworks.
- Soft Skills: Communication, teamwork, problem-solving, and adaptability are crucial for successful collaboration and project management.
Interview Questions and Answers
Technical Questions
Question 1: Explain the difference between JDK, JRE, and JVM.
-
Answer:
- JDK (Java Development Kit): A software development environment used for developing Java applications. It includes the JRE, an interpreter/loader (Java), a compiler (javac), an archiver (jar), a documentation generator (Javadoc), and other tools needed for Java development.
- JRE (Java Runtime Environment): A set of software tools for the development of Java applications. It provides the libraries, Java Virtual Machine (JVM), and other components to run applications written in Java.
- JVM (Java Virtual Machine): An abstract machine that enables your computer to run a Java program. It converts Java bytecode into machine language, allowing the program to be executed. It’s platform-independent and ensures that Java applications can run on any device that has the JVM installed.
-
Examples and Context:
- Development: When developing Java applications, you need the JDK. It contains tools for writing and testing your code.
- Execution: When you want to run a Java application, the JRE is sufficient. It provides the JVM and necessary libraries.
-
Real-World Scenario: Consider a scenario where you are tasked with setting up a development environment on a new machine. You would need to install the JDK. If you’re deploying a Java application on a server, the JRE would be required.
-
Common Pitfalls:
- Mistaking the JDK for JRE: Developers sometimes mistakenly believe that installing the JRE is sufficient for development, leading to missing development tools.
- Compatibility Issues: Ensure that the version of JDK/JRE matches the application’s requirements to avoid compatibility issues.
-
Follow-Up Points:
- How does JVM handle garbage collection?
- What are the different types of JDK available (like OpenJDK vs Oracle JDK)?
Question 2: What is the role of the ‘static’ keyword in Java?
-
Answer: The
static
keyword in Java is used for memory management primarily. It can be applied to variables, methods, blocks, and nested classes. When a member is declared static, it can be accessed without creating an instance of the class. -
Examples and Context:
- Static Variables: A static variable is shared among all instances of a class. For example,
static int count
keeps track of the number of instances created. - Static Methods: Static methods belong to the class rather than any object instance and can be called without creating an instance of the class. For example,
Math.max()
. - Static Blocks: Used for static initializations of a class. This block of code runs once when the class is loaded.
- Static Variables: A static variable is shared among all instances of a class. For example,
-
Real-World Scenario: Imagine a counter that tracks the number of instances of a class. Using a static variable, you can ensure that all instances share the same counter value, incremented each time an instance is created.
-
Reasoning Behind Best Practices:
- Use static for constants: Variables that do not change should be declared static to prevent unnecessary memory usage.
- Keep utility methods static: Methods that do not require any data from an instance of the class (like helper methods) should be static.
-
Pitfalls to Avoid:
- Overusing Static: Excessive use of static can lead to code that is difficult to test and maintain. Static members cannot be overridden, which can impact polymorphism.
- Thread Safety: Static variables are shared across all threads, which can lead to concurrency issues if not handled correctly.
-
Follow-Up Points:
- How does static affect inheritance?
- Can we override static methods? Why or why not?
Question 3: Describe the concept of exception handling in Java.
-
Answer: Exception handling in Java is managed via five keywords:
try
,catch
,finally
,throw
, andthrows
. It is used to handle runtime errors, allowing the normal flow of the program to continue. -
Examples and Context:
- try-catch: Enclose code that might throw an exception within a
try
block followed by one or morecatch
blocks to handle specific exceptions. - finally: A block that executes after the try-catch block, regardless of whether an exception was thrown. It’s typically used for cleanup activities.
- throw: Used to explicitly throw an exception.
- throws: Declares that a method can throw exceptions. It’s part of the method signature.
- try-catch: Enclose code that might throw an exception within a
-
Real-World Scenario: Consider a file reading operation. You would use a try-catch block to handle
IOException
that might occur if the file does not exist or cannot be read. -
Reasoning Behind Best Practices:
- Specific Exceptions: Catch specific exceptions rather than a generic
Exception
to handle different error conditions appropriately. - Resource Management: Use try-with-resources for managing resources like file streams to ensure they are closed properly.
- Specific Exceptions: Catch specific exceptions rather than a generic
-
Pitfalls to Avoid:
- Ignoring Exceptions: Catching exceptions and doing nothing with them can hide errors and make debugging difficult.
- Overusing Exceptions: Using exceptions for control flow can lead to performance issues.
-
Follow-Up Points:
- Difference between checked and unchecked exceptions?
- What happens if a try block does not have a catch block following it?
Behavioral Questions
Question 4: Describe a time when you had to work in a team to complete a project.
-
Answer: Teamwork is crucial in software development, as complex projects often require collaboration across different skill sets.
-
Example and Context:
- Scenario: During a previous role, I was part of a team tasked with developing a new feature for a financial application. My role was to integrate the Java-based backend with the front-end UI.
- Approach: We began by holding a series of planning meetings to divide the project into manageable tasks. Each team member was assigned specific responsibilities based on their expertise.
- Outcome: By maintaining open communication and using tools like Jira for task management, we completed the project ahead of schedule. The feature was well-received by users, increasing customer satisfaction.
-
Reasoning Behind Best Practices:
- Clear Communication: Regular updates and discussions help align team members and prevent misunderstandings.
- Task Delegation: Assigning tasks based on individual strengths ensures efficiency and quality.
-
Pitfalls to Avoid:
- Poor Coordination: Lack of coordination can lead to duplicate work or integration issues.
- Ignoring Team Input: Not valuing team members’ input can lead to low morale and missed opportunities for improvement.
-
Follow-Up Points:
- How do you handle conflicts in a team?
- What tools do you use for team collaboration?
Question 5: How do you prioritize tasks when working on multiple projects?
-
Answer: Prioritization is key to managing workload effectively, especially when juggling multiple projects.
-
Example and Context:
- Scenario: In a previous position, I was handling maintenance for an existing application while developing a new module for another project.
- Approach: I used the Eisenhower Box to categorize tasks into urgent/important. This helped me focus on tasks that aligned with business priorities and deadlines. Daily stand-ups were used to reassess priorities and adjust as necessary.
- Outcome: By maintaining a structured task list and adjusting as needed, I was able to deliver both projects on time without compromising quality.
-
Reasoning Behind Best Practices:
- Use of Priority Matrices: Helps in categorizing tasks and focusing on high-impact activities.
- Regular Re-evaluation: Priorities can shift; regularly reassessing ensures alignment with business needs.
-
Pitfalls to Avoid:
- Ignoring Low-Priority Tasks: They can accumulate and become urgent if not addressed in a timely manner.
- Over-commitment: Taking on too much can lead to burnout and decreased productivity.
-
Follow-Up Points:
- What tools do you use for task management?
- How do you handle conflicting deadlines?
Situational Questions
Question 6: How would you handle a situation where a critical piece of code in production is failing?
-
Answer: Handling production issues requires a calm, methodical approach to minimize impact and resolve the issue efficiently.
-
Example and Context:
- Scenario: A critical API endpoint was returning errors, affecting users’ ability to access essential services.
- Approach: First, I assessed the severity and impact of the issue to prioritize it accordingly. I then gathered logs and diagnostic information to identify the root cause. Once identified, I implemented a temporary fix to restore service while working on a permanent solution.
- Outcome: By acting swiftly and communicating with stakeholders, the issue was resolved with minimal downtime, and a post-mortem analysis was conducted to prevent future occurrences.
-
Reasoning Behind Best Practices:
- Immediate Assessment: Determine the impact and prioritize response based on severity.
- Communication: Keep stakeholders informed throughout the resolution process to manage expectations.
-
Pitfalls to Avoid:
- Panic and Rushed Fixes: Can lead to further issues. A calm, structured approach is essential.
- Lack of Documentation: Failing to document the incident and solution can hinder future troubleshooting efforts.
-
Follow-Up Points:
- What steps do you take to ensure similar issues don’t recur?
- How do you handle communication during a critical incident?
Question 7: Imagine you’re tasked with choosing a new technology stack for a project. How would you approach this decision?
-
Answer: Selecting a technology stack requires careful consideration of project requirements, team expertise, and future scalability.
-
Example and Context:
- Scenario: I was once responsible for recommending a tech stack for a greenfield project focused on real-time data processing.
- Approach: I began by gathering requirements and constraints, such as performance needs, budget, and existing infrastructure compatibility. I evaluated several options based on criteria like community support, documentation, and scalability. I also conducted proof-of-concept tests to assess real-world performance.
- Outcome: The chosen stack met all project requirements and allowed the team to deliver a performant, scalable application on time.
-
Reasoning Behind Best Practices:
- Requirements Analysis: Ensures that the chosen stack aligns with project goals and constraints.
- Scalability Considerations: Future growth should be factored into the decision to avoid costly migrations later.
-
Pitfalls to Avoid:
- Focusing Solely on Trends: Popularity does not equate to suitability for a specific project.
- Ignoring Team Expertise: A stack that the team is unfamiliar with can lead to steep learning curves and delays.
-
Follow-Up Points:
- How do you keep up with new technologies and decide when to adopt them?
- What factors would lead you to reconsider a chosen technology stack?
Problem-Solving Questions
Question 8: How do you approach debugging a complex issue in a Java application?
-
Answer: Debugging is a critical skill in development, requiring a systematic approach to isolate and resolve issues.
-
Example and Context:
- Scenario: A Java application was intermittently crashing, and the logs did not provide clear insights.
- Approach: I started by reproducing the issue consistently to understand the conditions under which it occurred. I used Java debuggers and profilers such as JDB or VisualVM to analyze the application’s runtime behavior. I also reviewed recent code changes to identify potential sources of the problem.
- Outcome: By narrowing down the issue to a specific module and using a process of elimination, I identified a memory leak caused by an unclosed resource. Fixing this resolved the crashes.
-
Reasoning Behind Best Practices:
- Reproduce the Issue: Consistent reproduction is key to understanding and diagnosing the problem.
- Divide and Conquer: Isolate sections of code to identify the problematic area.
-
Pitfalls to Avoid:
- Assumptions: Avoid assuming the cause without evidence, which can lead to wasted effort.
- Ignoring Logs: Logs can provide critical insights; overlooking them can delay resolution.
-
Follow-Up Points:
- What tools do you find most effective for debugging?
- How do you ensure issues do not reoccur?
Question 9: Explain how you would optimize a slow-performing Java application.
-
Answer: Optimization involves identifying bottlenecks and improving performance through various strategies.
-
Example and Context:
- Scenario: A Java application was experiencing slow response times during peak usage.
- Approach: I began by profiling the application to identify bottlenecks. I focused on optimizing database queries, reducing unnecessary computations, and implementing caching where appropriate. I also reviewed and improved the use of collections and data structures to ensure efficient operations.
- Outcome: These optimizations resulted in a significant improvement in response times, enhancing user satisfaction and reducing server load.
-
Reasoning Behind Best Practices:
- Profiling First: Before making changes, identify where the real bottlenecks are to avoid unnecessary optimizations.
- Efficient Data Structures: Use the right data structures to improve performance in terms of time complexity.
-
Pitfalls to Avoid:
- Premature Optimization: Focus on optimizing code that truly impacts performance rather than micro-optimizations.
- Ignoring Scalability: Ensure that optimizations do not compromise the application’s ability to scale.
-
Follow-Up Points:
- What metrics do you use to measure performance improvements?
- How do you balance performance with maintainability?
Question 10: How would you handle a situation where a new feature request conflicts with existing functionality?
-
Answer: Balancing new feature requests with existing functionality requires careful consideration and stakeholder communication.
-
Example and Context:
- Scenario: A new feature request required changes to a core algorithm that other features depended on.
- Approach: I analyzed the impact of the requested changes on existing functionality. I then discussed with stakeholders to understand the priority and necessity of the new feature. I explored alternative implementations that could achieve the desired outcome with minimal disruption. Prototyping and testing ensured that changes met all requirements.
- Outcome: By finding a compromise that met both new and existing requirements, the feature was successfully integrated without negative impacts on the application’s stability.
-
Reasoning Behind Best Practices:
- Impact Analysis: Understand the full scope of changes to avoid unintended consequences.
- Stakeholder Communication: Align with stakeholders on priorities and potential trade-offs.
-
Pitfalls to Avoid:
- Ignoring Dependencies: Overlooking dependencies can lead to widespread issues.
- Lack of Testing: Thorough testing is essential to ensure that changes do not introduce new bugs.
-
Follow-Up Points:
- How do you prioritize conflicting feature requests?
- What strategies do you use to mitigate risks when implementing new features?
This guide is designed to provide a comprehensive overview of the skills and scenarios you may encounter during a Java Developer interview. By preparing with these questions and considering multiple perspectives and solutions, you’ll be well-equipped to demonstrate your technical expertise and problem-solving abilities.
More Software Engineering Interview Guides
Explore more interview guides for Technical positions.
AI Engineer Interview Preparation
The AI Engineer Interview Preparation guide equips job seekers with essential skills and knowledge to excel in AI eng...
Back-End Developer Interview Help
Back-End Developer Interview Help equips job seekers with essential strategies and insights to ace their interviews. ...
Mobile App Developer Interview Help
The Mobile App Developer Interview Help guide equips job seekers with essential strategies to excel in interviews. Le...
Blockchain Developer Interview Questions and Answers
This guide offers a comprehensive collection of Blockchain Developer interview questions and answers, designed to hel...
Software Architect Interview Questions and Answers
Discover essential insights with our Software Architect Interview Questions and Answers guide. Tailored for job seeke...
Recent Blog Articles
Check out recent articles from Tustin Recruiting on all things hiring.
How to Implement Structured JSON-LD for Google Jobs
Learn how to implement structured JSON-LD for Google Jobs to improve your job postings and attract more qualified can...
Common Employee Benefits in Orange County, CA Private Sector
Discover common employee benefits offered by private sector employers in Orange County, CA.
10 High-Paying Sales Jobs You Can Get Without a Degree
Discover 10 high-paying sales jobs you can get without a degree, including entry-level roles and opportunities for ca...
When to Follow Up with a Recruiter
Learn when to follow up with a recruiter after submitting your resume and when to wait for best practices.
Exceptional Software Engineer Jobs in Orange County
Discover top software engineer jobs in Orange County. Unlock salary insights, skills needed, and career tips.
Featured Jobs
-
- Company
- Tustin Recruiting
- Title and Location
- Account Executive Equipment Finance
- Irvine, CA
- Employment Type
- FULL_TIME
- Salary
- $75,000-$95,000/YEAR
- Team and Date
- Equipment Finance
- Posted: 02/09/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Account Executive Equipment Finance
- Anaheim Hills, CA
- Employment Type
- FULL_TIME
- Salary
- $75,000-$95,000/YEAR
- Team and Date
- Equipment Finance
- Posted: 02/09/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Junior Account Executive
- Hayward, CA
- Employment Type
- FULL_TIME
- Salary
- $62,330-$79,329/YEAR
- Team and Date
- Software
- Posted: 01/29/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Sales Operations Coordinator
- Eugene, OR
- Employment Type
- FULL_TIME
- Salary
- $45,156-$58,201/YEAR
- Team and Date
- Software
- Posted: 01/29/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Account Executive
- Cypress, TX
- Employment Type
- FULL_TIME
- Salary
- $55,000-$70,000/YEAR
- Team and Date
- Equipment Finance
- Posted: 01/29/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Mobile App Developer
- Lakewood, CA
- Employment Type
- FULL_TIME
- Salary
- $85,013-$118,074/YEAR
- Team and Date
- Software
- Posted: 01/29/2025
Ready to find your next great hire?
Let's discuss your hiring needs. With our deep Orange County network and 20+ years of experience, we'll help you find the perfect candidate.
20+ Years Experience
Deep expertise and a proven track record of successful placements.
Direct-Hire Focus
Specialized in permanent placements that strengthen your team for the long term.
Local Market Knowledge
Unmatched understanding of Orange County's talent landscape and salary expectations.
Premium Job Board
Access top Orange County talent through our curated job board focused on quality over quantity.
Tustin Recruiting is for Everyone
At Tustin Recruiting, we are dedicated to fostering an inclusive environment that values diverse perspectives, ideas, and backgrounds. We strive to ensure equal employment opportunities for all applicants and employees. Our commitment is to prevent discrimination based on any protected characteristic, including race, color, ancestry, national origin, religion, creed, age, disability (mental and physical), sex, gender, sexual orientation, gender identity, gender expression, medical condition, genetic information, family care or medical leave status, marital status, domestic partner status, and military and veteran status.
We uphold all characteristics protected by US federal, state, and local laws, as well as the laws of the country or jurisdiction where you work.