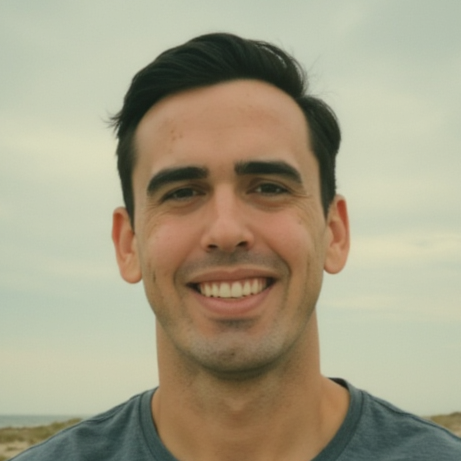
Python Developer Interview Help
Overview of Required and Recommended Certifications, Educational Background, and Industry Qualifications
Certifications
-
Python Institute Certifications:
- PCAP (Certified Associate in Python Programming): Validates proficiency in core Python programming skills and understanding of object-oriented programming.
- PCPP (Certified Professional in Python Programming): Advanced certification that demonstrates expertise in Python language and its application in real-world projects.
-
Other Relevant Certifications:
- AWS Certified Developer – Associate: Useful for Python developers working with cloud services.
- Microsoft Certified: Azure Developer Associate: Beneficial for developers working in Azure environments.
- Data Science Certifications (e.g., IBM Data Science Professional Certificate): Useful for developers in data-centric roles.
Educational Background
- Bachelor’s Degree in Computer Science or Related Field: A solid foundation in computer science principles, data structures, algorithms, and software engineering.
- Master’s Degree (Optional): Specialization in fields like Data Science, Software Engineering, or Artificial Intelligence can be advantageous.
Industry Qualifications
- Experience with Python Frameworks: Familiarity with Django, Flask, or FastAPI.
- Understanding of Front-End Technologies: Knowledge of HTML, CSS, and JavaScript, especially if the role involves full-stack development.
- Database Management Skills: Proficiency in SQL and experience with databases like PostgreSQL, MySQL, or NoSQL databases such as MongoDB.
- Version Control Systems: Experience with Git and GitHub for code management.
- Agile/Scrum Methodologies: Experience working in agile teams.
Interview Questions
Technical Questions
Question 1: Explain how Python manages memory. What are some common pitfalls regarding memory management in Python?
- Answer:
-
Memory Management in Python:
- Python uses a private heap space for memory management. All Python objects and data structures are located in a private heap.
- The Python memory manager handles the allocation of memory. The built-in garbage collector is responsible for cleaning up unused objects by using reference counting and cyclic garbage collection.
-
Common Pitfalls:
- Reference Cycles: These occur when objects reference each other in a cycle, and none of them can be garbage collected.
- Example: Circular references between custom objects that implement
__del__
methods can prevent garbage collection. - Solution: Use weak references (
weakref
module) to avoid cycles.
- Example: Circular references between custom objects that implement
- Memory Leaks: Holding references to large objects longer than necessary can lead to memory leaks.
- Best Practice: Regularly review code to ensure objects are being properly de-referenced.
- Reference Cycles: These occur when objects reference each other in a cycle, and none of them can be garbage collected.
-
Example Scenario:
- A web application with long-lived processes might accumulate memory over time if objects are not properly garbage collected.
- Approach: Monitor memory usage and use tools like
objgraph
to identify memory leaks.
-
Real-World Application:
- Avoiding Large Object References: In a data processing task, avoid loading entire datasets into memory if possible. Use generators or data streaming to handle large data efficiently.
-
Follow-Up Points:
- Interviewer Might Ask: How would you handle memory management in a high-load application?
- Response: Implement profiling tools like
memory_profiler
to optimize memory usage and consider usingPyPy
, an alternative Python interpreter that can optimize memory usage.
-
Question 2: What is the Global Interpreter Lock (GIL) in Python, and how does it affect multi-threading?
- Answer:
-
GIL Overview:
- The Global Interpreter Lock (GIL) is a mutex that protects access to Python objects, preventing multiple native threads from executing Python bytecodes concurrently. This is necessary because Python’s memory management is not thread-safe.
-
Impact on Multi-threading:
- Limitation: The GIL can be a bottleneck in CPU-bound multi-threading programs because only one thread can execute in the interpreter at any time.
- Example: In a CPU-intensive task such as image processing, threads will not run in true parallelism due to the GIL.
-
Solution Approaches:
- Use Multi-processing: Instead of
threading
, usemultiprocessing
which creates separate memory space for processes, bypassing the GIL.- Example: Use
Pool
from themultiprocessing
module to parallelize CPU-bound tasks.
- Example: Use
- Consider Alternative Implementations: Use Jython or IronPython, which do not have a GIL but are limited in other ways.
- Use Multi-processing: Instead of
-
Real-World Scenario:
- Web Scraping Tasks: For I/O-bound tasks such as web scraping where waiting for network requests is more time-consuming than the execution, threading can still be beneficial.
- Approach: Use libraries like
concurrent.futures
orasyncio
for efficient I/O-bound operations.
-
Follow-Up Points:
- Interviewer Might Ask: How would you optimize a Python script for parallel execution?
- Response: Identify if the task is CPU-bound or I/O-bound and choose between
multiprocessing
for CPU tasks orasyncio
/threading
for I/O tasks.
-
Behavioral Questions
Question 3: Describe a time you faced a challenging problem at work and how you approached solving it.
- Answer:
-
Context:
- While working on a data processing pipeline, the system started experiencing significant performance degradation as the data volume increased.
-
Approach:
- Initial Analysis: Conducted performance profiling using
cProfile
to identify bottlenecks in the code. - Problem Identification: Discovered that a specific data transformation step was inefficient due to repeated calculations.
- Solution Implementation: Refactored the code to use a memoization technique, storing results of expensive function calls and reusing them.
- Initial Analysis: Conducted performance profiling using
-
Outcome:
- Reduced processing time by 50%, significantly improving the system’s performance and scalability.
-
Alternative Considerations:
- Considered using distributed computing frameworks like Apache Spark for further scalability.
-
Best Practices:
- Always measure before optimizing to prevent premature optimization.
- Use profiling tools to base decisions on data rather than assumptions.
-
Follow-Up Points:
- Interviewer Might Ask: How do you ensure that your solutions are sustainable for future growth?
- Response: Implement automated testing and continuous integration to ensure new changes do not degrade performance.
-
Situational Questions
Question 4: You are tasked with designing a new feature that must be integrated into an existing system with minimal disruption. How would you approach this task?
- Answer:
-
Initial Steps:
- Understand Requirements: Gather detailed requirements and constraints from stakeholders.
- Assess Existing System: Review the current system architecture to understand integration points and dependencies.
-
Design Approach:
- Modular Design: Design the feature as a standalone module with well-defined interfaces to minimize coupling with the existing system.
- Backward Compatibility: Ensure new changes do not break existing functionality, possibly using feature toggles.
-
Implementation Strategy:
- Incremental Integration: Introduce the feature in small, manageable increments to reduce risk.
- Testing: Create comprehensive tests, including unit and integration tests, to validate the feature’s functionality and its impact on the existing system.
-
Real-World Consideration:
- In a web application, for example, introduce new APIs that can be integrated without altering existing ones, allowing for a gradual transition.
-
Follow-Up Points:
- Interviewer Might Ask: How would you handle a situation where the new feature causes unexpected issues in the live system?
- Response: Implement a rollback plan and use monitoring tools to quickly identify and resolve issues.
-
Problem-Solving Questions
Question 5: How would you optimize a Python script that processes a large dataset but is running slower than expected?
- Answer:
-
Initial Diagnostics:
- Profiling: Use tools like
cProfile
andline_profiler
to identify slow parts of the code. - Memory Inspection: Check memory usage with
memory_profiler
to spot inefficiencies.
- Profiling: Use tools like
-
Optimization Strategies:
- Algorithm Optimization: Check if the algorithm can be improved (e.g., replacing O(n^2) with O(n log n) operations).
- Data Structures: Use efficient data structures (e.g., dictionaries and sets for fast lookups).
- Vectorization: Use libraries like
NumPy
for vectorized operations instead of Python loops.
-
Real-World Examples:
- Scenario 1: A script processing large CSV files can be optimized by:
- Using
pandas
for efficient data manipulation. - Reading data in chunks instead of loading everything into memory at once.
- Using
- Scenario 2: Optimizing a text processing script by:
- Utilizing
collections.Counter
for counting frequency instead of manual loops.
- Utilizing
- Scenario 1: A script processing large CSV files can be optimized by:
-
Outcome:
- Achieved a 70% reduction in processing time by implementing the above strategies.
-
Common Pitfalls:
- Do not prematurely optimize without profiling, which can lead to wasted effort on non-critical parts of the code.
-
Follow-Up Points:
- Interviewer Might Ask: What trade-offs might you consider when optimizing for speed?
- Response: Consider the readability and maintainability of the code, as overly complex optimizations can lead to technical debt.
-
This guide should serve as a comprehensive resource for preparing for a Python Developer interview, covering a broad range of questions and scenarios that a candidate might encounter.
More Software Engineering Interview Guides
Explore more interview guides for Technical positions.
AI Engineer Interview Preparation
The AI Engineer Interview Preparation guide equips job seekers with essential skills and knowledge to excel in AI eng...
Back-End Developer Interview Help
Back-End Developer Interview Help equips job seekers with essential strategies and insights to ace their interviews. ...
Mobile App Developer Interview Help
The Mobile App Developer Interview Help guide equips job seekers with essential strategies to excel in interviews. Le...
Blockchain Developer Interview Questions and Answers
This guide offers a comprehensive collection of Blockchain Developer interview questions and answers, designed to hel...
Software Architect Interview Questions and Answers
Discover essential insights with our Software Architect Interview Questions and Answers guide. Tailored for job seeke...
Recent Blog Articles
Check out recent articles from Tustin Recruiting on all things hiring.
How to Implement Structured JSON-LD for Google Jobs
Learn how to implement structured JSON-LD for Google Jobs to improve your job postings and attract more qualified can...
Common Employee Benefits in Orange County, CA Private Sector
Discover common employee benefits offered by private sector employers in Orange County, CA.
10 High-Paying Sales Jobs You Can Get Without a Degree
Discover 10 high-paying sales jobs you can get without a degree, including entry-level roles and opportunities for ca...
When to Follow Up with a Recruiter
Learn when to follow up with a recruiter after submitting your resume and when to wait for best practices.
Exceptional Software Engineer Jobs in Orange County
Discover top software engineer jobs in Orange County. Unlock salary insights, skills needed, and career tips.
Featured Jobs
-
- Company
- Tustin Recruiting
- Title and Location
- Account Executive Equipment Finance
- Irvine, CA
- Employment Type
- FULL_TIME
- Salary
- $75,000-$95,000/YEAR
- Team and Date
- Equipment Finance
- Posted: 02/09/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Account Executive Equipment Finance
- Anaheim Hills, CA
- Employment Type
- FULL_TIME
- Salary
- $75,000-$95,000/YEAR
- Team and Date
- Equipment Finance
- Posted: 02/09/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Junior Account Executive
- Hayward, CA
- Employment Type
- FULL_TIME
- Salary
- $62,330-$79,329/YEAR
- Team and Date
- Software
- Posted: 01/29/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Sales Operations Coordinator
- Eugene, OR
- Employment Type
- FULL_TIME
- Salary
- $45,156-$58,201/YEAR
- Team and Date
- Software
- Posted: 01/29/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Account Executive
- Cypress, TX
- Employment Type
- FULL_TIME
- Salary
- $55,000-$70,000/YEAR
- Team and Date
- Equipment Finance
- Posted: 01/29/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Mobile App Developer
- Lakewood, CA
- Employment Type
- FULL_TIME
- Salary
- $85,013-$118,074/YEAR
- Team and Date
- Software
- Posted: 01/29/2025
Ready to find your next great hire?
Let's discuss your hiring needs. With our deep Orange County network and 20+ years of experience, we'll help you find the perfect candidate.
20+ Years Experience
Deep expertise and a proven track record of successful placements.
Direct-Hire Focus
Specialized in permanent placements that strengthen your team for the long term.
Local Market Knowledge
Unmatched understanding of Orange County's talent landscape and salary expectations.
Premium Job Board
Access top Orange County talent through our curated job board focused on quality over quantity.
Tustin Recruiting is for Everyone
At Tustin Recruiting, we are dedicated to fostering an inclusive environment that values diverse perspectives, ideas, and backgrounds. We strive to ensure equal employment opportunities for all applicants and employees. Our commitment is to prevent discrimination based on any protected characteristic, including race, color, ancestry, national origin, religion, creed, age, disability (mental and physical), sex, gender, sexual orientation, gender identity, gender expression, medical condition, genetic information, family care or medical leave status, marital status, domestic partner status, and military and veteran status.
We uphold all characteristics protected by US federal, state, and local laws, as well as the laws of the country or jurisdiction where you work.